SpringBoot整合MybaitsPlus
发表于更新于
字数总计:986阅读时长:5分钟阅读量: 成都
创建项目
使用Intellj IDEA创建SpringBoot项目,其中Server URL可以使用阿里云的URL,地址为https://start.aliyun.com/
,填写完毕后点击Next
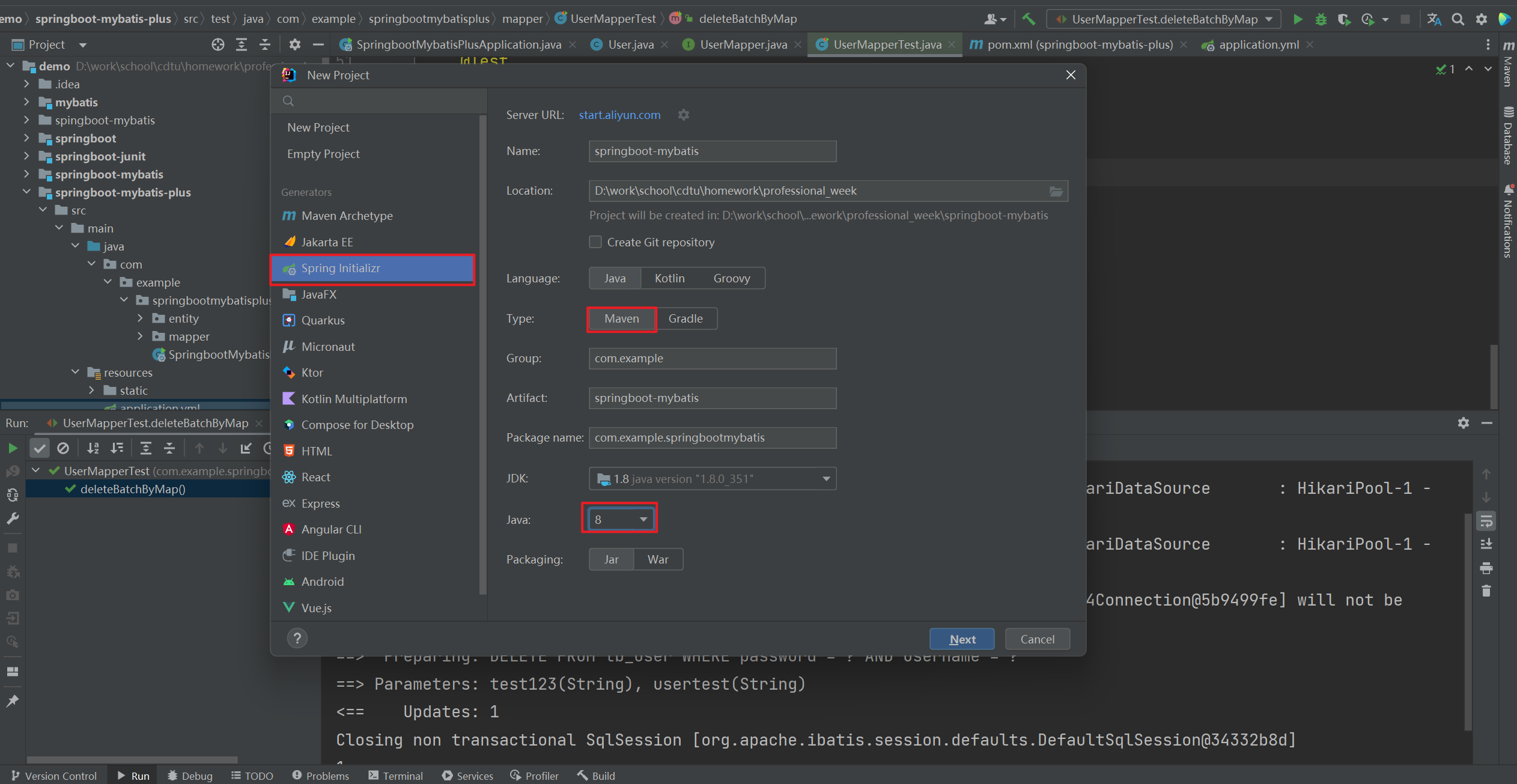
选择3.0以下的SpringBoot版本,并引入SpringWeb依赖,点击Create创建项目

配置
导入坐标
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.26</version> </dependency>
<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency>
<dependency> <groupId>com.baomidou</groupId> <artifactId>mybatis-plus-boot-starter</artifactId> <version>3.4.0</version> </dependency>
<dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.2.2</version> </dependency>
|
数据源
在application.yml
文件中写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| server: port: 8080 spring: datasource: url: jdbc:mysql://127.0.0.1:3307/itheima?useSSL=false username: manager password: mk123456 driver-class-name: com.mysql.jdbc.Driver
mybatis-plus: global-config: db-config: table-prefix: tb_ id-type: auto configuration: map-underscore-to-camel-case: true log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
mybatis: type-aliases-package: com.example.springbootmybatisplus.entity mapper-locations: classpath*:mapper/*.xml
|
建表
执行下面的sql脚本,创建测试用的表
1 2 3 4 5 6 7 8 9 10 11 12 13
| drop table if exists tb_user;
create table tb_user( id int primary key auto_increment, username varchar(20), password varchar(20), gender char(1), addr varchar(30) );
INSERT INTO tb_user VALUES (1, 'zhangsan', '123', '男', '北京'); INSERT INTO tb_user VALUES (2, '李四', '234', '女', '天津'); INSERT INTO tb_user VALUES (3, '王五', '11', '男', '西安');
|
实体类
新建实体类com.example.springbootmybatisplus.entity.User
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.example.springbootmybatisplus.entity;
import com.baomidou.mybatisplus.annotation.TableId; import com.baomidou.mybatisplus.annotation.TableName; import lombok.Data;
@Data @TableName("tb_user") public class User { @TableId() private Integer id; private String username; private String password; private Character gender; private String addr; }
|
映射
注解映射
新建Mapper接口com.example.springbootmybatisplus.mapper.UserMapper
1 2 3 4 5 6 7 8 9
| package com.example.springbootmybatisplus.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper; import com.example.springbootmybatisplus.entity.User; import org.apache.ibatis.annotations.Mapper;
@Mapper public interface UserMapper extends BaseMapper<User> { }
|
XML映射
在src/resources/mapper
下创建UserMapper.xml
文件,模板如下
1 2 3 4 5 6 7
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.springbootmybatisplus.mapper.UserMapper">
</mapper>
|
配置扫描
在启动类加上@MapperScan({"com.example.springbootmybatisplus.mapper"})
以配置Mapper扫描
1 2 3 4 5 6 7 8 9
| @SpringBootApplication @MapperScan({"com.example.springbootmybatisplus.mapper"}) public class SpringbootmybatisplusApplication {
public static void main(String[] args) { SpringApplication.run(SpringbootmybatisplusApplication.class, args); }
}
|
服务类
新建Service接口com.example.springbootmybatisplus.service.UserService
1 2 3 4 5 6 7
| package com.example.springbootmybatisplus.service;
import com.example.springbootmybatisplus.entity.User; import com.baomidou.mybatisplus.extension.service.IService;
public interface UserService extends IService<User> { }
|
新建Service实现类com.example.springbootmybatisplus.service.impl.UserServiceImpl
1 2 3 4 5 6 7 8 9 10 11
| package com.example.springbootmybatisplus.service.impl;
import com.example.springbootmybatisplus.entity.User; import com.example.springbootmybatisplus.mapper.UserMapper; import com.example.springbootmybatisplus.service.UserService; import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; import org.springframework.stereotype.Service;
@Service public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService { }
|
控制器
新建Controller控制器com.example.springbootmybatisplus.controller.UserController
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| package com.example.springbootmybatisplus.controller;
import com.example.springbootmybatisplus.entity.User; import com.example.springbootmybatisplus.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import java.util.List;
@RestController @RequestMapping("/user") public class UserController { @Autowired private UserService userService;
@GetMapping public List<User> findAll() { return userService.list(); } }
|
测试
运行启动类,使用浏览器访问http://localhost:8080/user,返回值如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| [ { id: 1, username: "zhangsan", password: "123", gender: "男", addr: "北京" }, { id: 2, username: "李四", password: "234", gender: "女", addr: "天津" }, { id: 3, username: "王五", password: "11", gender: "男", addr: "西安" } ]
|